Longer Sessions
Keep longer sessions alive on disconnect.
Sessions will end when browsers disconnect.
You can keep sessions alive until they time out or until you request their release.
Once the expiresAt
time passes, the session will end.
Below, we are creating a session for a specified project:
You can see in the body of the request, we specify the projectId
located in our Dashboard’s Settings Tab.
In the next sections, we’ll walkthrough how to make the sessions stay alive longer.
How do Sessions End?
Sessions typically end when browsers disconnect. This is usually done by a timeout, which can be specified in the request body of the Session Creation.
All sessions have a Default Timeout that determines how long a session will stay alive.
You can set the default maximum duration for your project in Settings.
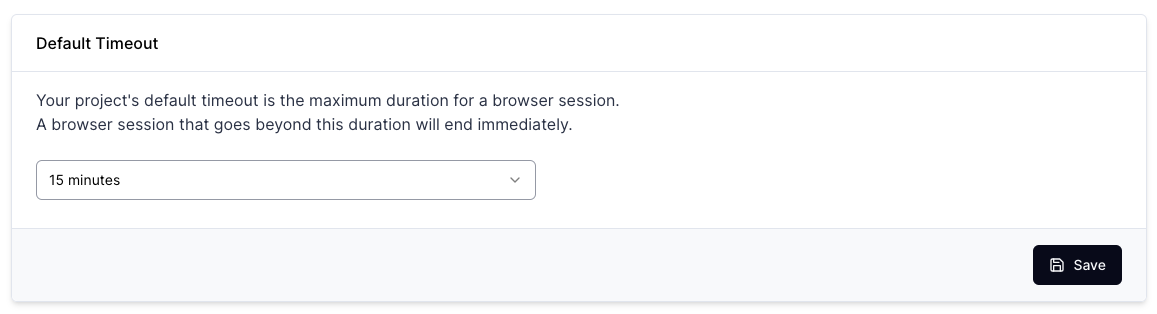
How do I customize the timeout in my project and per session?
To set a custom timeout per session, you can specify the timeout
option in the request body via the API.
Here, we’ve set the timeout to 3600 seconds (1 hour). This means that the session will stay alive until the specified timeout.
Additionally, if the browser disconnects and the session is not configured for keep-alive, it will end immediately. We explain this more in the next section.
How do I keep sessions alive between disconnects?
Browserbase offers a keepAlive
feature to keep sessions alive between disconnects.
In order to keep a session alive for longer than the default timeout, we need to reconnect after a session disconnect from the timeout.
This allows you to keep the session alive for as long as you want.
Setting keepAlive
to true
will reconnect to the session after timeout, as shown below:
Keep Alive functionality is only supported for those that are on the Startup plan.
Reconnecting to the session after timeout will keep the session alive for as long as you want.
When the session disconnects, the session will reconnect and perform as usual.
It’s important to know the automation tool (such as Playwright, Puppeteer, or Selenium) are responsible for disconnecting and reconnecting.
Once a session times out, it is over and cannot be used again.
How do I stop a session that is being kept alive?
Keeping a session alive means that the session will stay alive until you stop it manually or it times out.
In order to stop a session that is being kept alive, you can use the Browserbase API or SDK.
If you don’t stop sessions manually and just wait for them to timeout, you’ll be charged all the browser minutes used. It’s best practice to be efficient by stopping sessions when you’re done with them.
Why keep sessions alive?
Keeping sessions alive are important for things like completing tasks that take a long time to complete. It’s great for
Some Key Benefits of Keeping Sessions Alive:
- You can keep working on sessions without worrying about timeouts.
- Less reload times and faster navigation.
- Ensured network stability to avoid interrupting user workflows.
Was this page helpful?